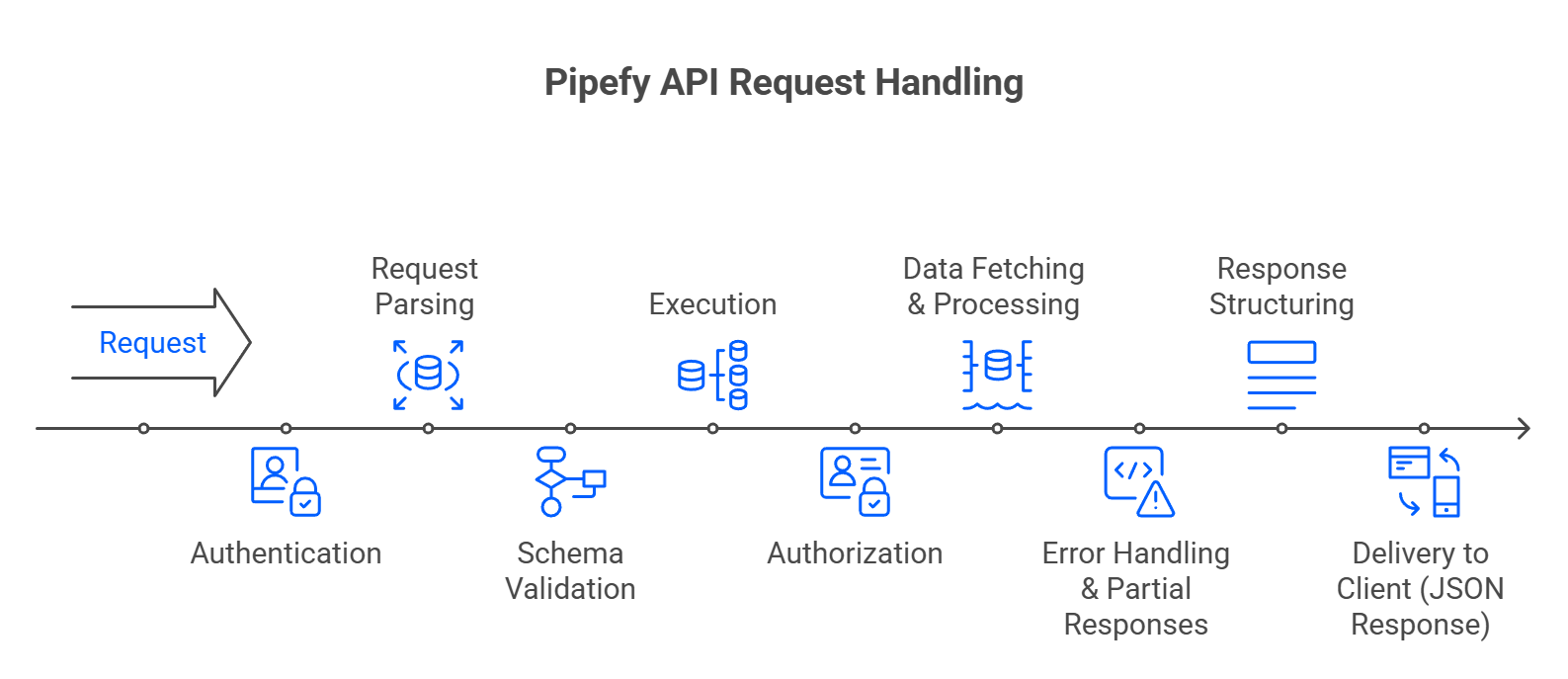
1. Authentication: Verify User Identity
When a request reaches Pipefy’s API, the system first confirms the user is logged in or has valid credentials (e.g., API token, SSO session). If authentication fails, the process stops immediately.
Example: An automation tool (like Zapier) sends a request to fetch cards from a pipe. If the API token is missing or expired, Pipefy returns an error like 401 Unauthorized
.
2. Request Parsing: Interpret the Query
The API reads the GraphQL query to understand "what" data is requested or "what" action to perform (e.g., fetching cards, updating fields). It breaks down the query’s structure, such as fields, arguments, and nested data.
Example: A query asking for cards in phase "Approval" with title and due_date
is parsed to identify which database tables or services to access.
3. Schema Validation: Check for Permitted Actions
Pipefy’s GraphQL schema acts as a rulebook. The system checks if the query is valid: Do the requested fields exist? Are arguments (like phaseId
) formatted correctly? Invalid requests are rejected here.
Example: A query trying to access a non-existent field like cardPriority
would return an error: Cannot query field 'cardPriority'
.
4. Execution: Resolve Data Layer-by-Layer
The API processes the query step-by-step, starting with root fields (e.g., cards
or pipes
) and moving to nested fields (e.g., phase
, assignees
). Each field has a "resolver" function that fetches its data.
Example: To get cards in the "Approval" phase, Pipefy first fetches the pipe, then its phases, then filters cards in that phase.
5. Authorization: Validate Access to Specific Data
Even if authenticated, users might lack permissions for certain data. Resolvers check roles or access rights at each step (e.g., can this user view cards in this pipe?).
Example: A member without “Admin” rights tries to fetch pipe settings
—the resolver blocks this field and returns null
with an error.
6. Data Fetching & Processing
Authorized resolvers fetch data from Pipefy’s database, external services, or connected apps (e.g., Salesforce). Complex queries might combine data from multiple sources.
Pipefy Example: Fetching a card’s due_date
from the main database and its attachments
from a cloud storage service like Google Drive.
7. Error Handling & Partial Responses
If part of the query fails (e.g., no access to a field), Pipefy omits that data but returns the rest. Errors are added to an errors array in the response for transparency.
Example: A request for title
, due_date
, and budget
returns title
and due_date
but skips budget with an error: "Cannot access restricted field"
.
8. Response Structuring
The final JSON response mirrors the shape of the original query, ensuring predictable results. Data is nested exactly as requested, with errors appended.
Example: A query for organizations { name, pipes { id, name } }
returns a list where each card’s title is paired with its phase name.
9. Delivery to Client
The API sends an HTTP response (status 200) containing the structured data and/or errors. Clients parse this to update UIs, trigger workflows, or display feedback.
Example: A connected dashboard displays cards from "Approval" phase.