card-badges
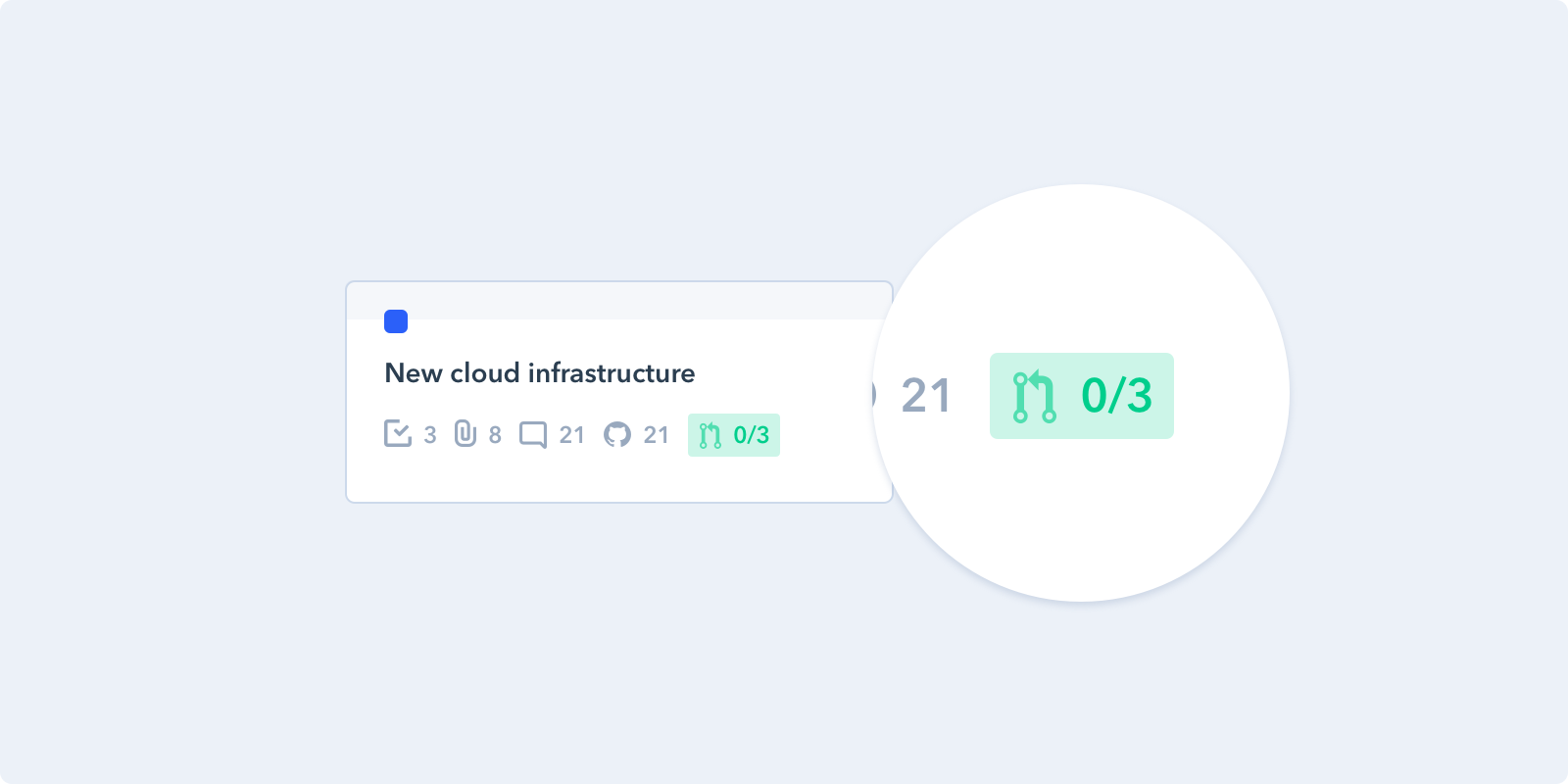
Github example app
Add badges to closed card, you can use the Live version when you need to get other card data, make external requests or need to have a refresh interval. You can use the Fixed version if you don't need that.
var getCountBadge = function(p, context){
return new Promise(function(resolve) {
p.cardAttachments().then(function(attachments) {
var claimed = attachments.filter(function(attachment){
return attachment.url.indexOf('https://github.com') === 0;
});
if (claimed && claimed.length) {
resolve({
text: claimed.length,
icon: ICON_GRAY,
title: claimed.length + " Github PRs attached to this card",
})
} else {
resolve(null)
}
});
});
};
PipefyApp.initCall({
'card-badges': function(p, context){
return [
// Sample Live Badge
{
live: getCountBadge,
refreshInterval: 20,
},
// Sample Fixed Badge
{
text: context.card.current_phase.name,
icon: 'https://cdn.glitch.com/03813ab1-4482-45be-b7f7-74e8948d7ae7%2Ficon-gray.svg?1505743926910',
title: 'Sample fixed badge',
}
];
},
});
Receive
- p: Pipefy Client
- context: Object with Pipe and Card (More details about Card object)
{
pipe: { id: '23dfu', name: 'Hotdog app' },
card: {
id: "233dfd",
title: "Build iOS App",
due_date: "...",
labels: [],
assignees: [],
}
}
Return
Expect to return an Array of card badges
Live
- live: Expect to return Promise that resolves a Card badge object, same options from Fixed example
- refreshInterval: Number of seconds to refresh and call live function. Minimum of 20 seconds
Fixed
- text: Text to be displayed inside badge
- icon: SVG relative path or full URL, svg fill color must be equal to #9AAABE
- color: Background colors, available options are
red
,yellow
,green
andblue
. - title: HTML Title that will be displayed when user hover the badge
Updated 4 months ago